## 数据表结构及关系  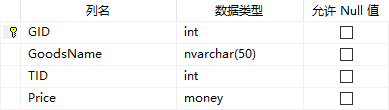 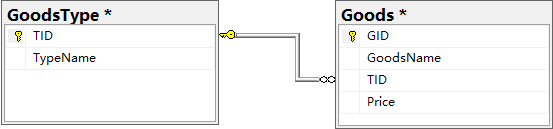 ## 创建数据库帮助类 ```csharp using System; using System.Data; using System.Data.SqlClient; using System.Windows.Forms; namespace TreeViewDemo { /// /// 数据库帮助类 /// public static class DBHelper { /// /// 连接字符串 /// private static string constr = @"server=.;database=Demo;user=sa;pwd=cssl#123"; /// /// 获取读取器,使用完毕时需要关闭读取器 /// /// 需要执行的SQL语句 /// 数据读取器 public static SqlDataReader ExecuteReader(string sql) { // 创建数据库连接对象,并指定连接字符串 SqlConnection con = new SqlConnection(constr); // 创建命令对象 SqlCommand cmd = new SqlCommand(sql, con); try { con.Open(); return cmd.ExecuteReader(CommandBehavior.CloseConnection); } catch (Exception ex) { MessageBox.Show($"访问数据库是发生意外,错误信息:\r\r{ex.Message}", "温馨提示", MessageBoxButtons.OK, MessageBoxIcon.Error); } return null; } } } ``` ## 窗体加载时,创建默认根节点 ```csharp /// /// 窗体加载时触发该事件 /// /// /// private void frmTreeViewDmeo_Load(object sender, EventArgs e) { BindRootNode(); //绑定根节点 tvDemo.ExpandAll(); //展开所有节点 } /// /// 绑定根节点 /// private void BindRootNode() { TreeNode tn = new TreeNode(); tn.Tag = 0; //绑定隐藏数据 tn.Text = "全部商品"; tvDemo.Nodes.Add(tn); //将创建出来的节点添加到TreeView控件中 } ``` 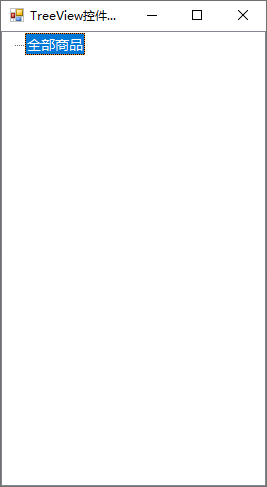 ## 绑定二级节点-商品分类 在 `BindRootNode()`方法最末尾添加如下代码: ```csharp BindGoodsType(tn); //绑定商品分类,将商品分类添加到"全部商品"节点中 ``` `BindGoodsType(TreeNode tn)`方法定义如下: ```csharp /// /// 加载商品分类 /// /// 根节点 private void BindGoodsType(TreeNode tn) { // 根节点创建后,绑定一级目录 [商品分类] string getGoodsTypeInfo = $"SELECT TID,TypeName FROM GoodsType"; SqlDataReader r = DBHelper.ExecuteReader(getGoodsTypeInfo); while (r != null && r.HasRows && r.Read()) { TreeNode typeNode = new TreeNode(); typeNode.Text = r["TypeName"].ToString(); typeNode.Tag = r["TID"]; tn.Nodes.Add(typeNode); } if (r != null) r.Close(); } ``` 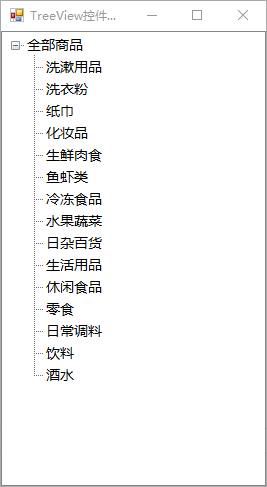 ## 加载不同分类下的不同商品信息 在方法 `BindGoodsType(TreeNode tn)`里的 `while`循环中最末尾添加如下代码: ```csharp // 在绑定该分类后,立马绑定当前分类下所有商品信息 BindGoodsInfo(typeNode); ``` `BindGoodsInfo(TreeNode typeNode)`方法定义如下: ```csharp /// /// 加载指定分类的商品信息 /// /// 商品分类节点 private void BindGoodsInfo(TreeNode typeNode) { string getGoodsTypeInfo = $"SELECT GID,GoodsName,Price FROM Goods WHERE TID = {typeNode.Tag}"; SqlDataReader r = DBHelper.ExecuteReader(getGoodsTypeInfo); while (r != null && r.HasRows && r.Read()) { TreeNode goodsNode = new TreeNode(); goodsNode.Text = $"{r["GoodsName"]} - {r["Price"]}元"; goodsNode.Tag = r["GID"]; typeNode.Nodes.Add(goodsNode); } if (r != null) r.Close(); } ``` 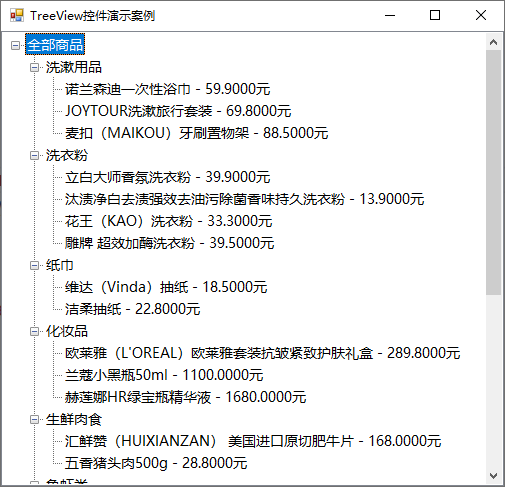 ## 完整代码展示 ```csharp using System; using System.Windows.Forms; using System.Data.SqlClient; namespace TreeViewDemo { public partial class frmTreeViewDmeo : Form { public frmTreeViewDmeo() { InitializeComponent(); } /// /// 窗体加载时触发该事件 /// /// /// private void frmTreeViewDmeo_Load(object sender, EventArgs e) { BindRootNode(); //绑定根节点 tvDemo.ExpandAll(); //展开所有节点 } /// /// 绑定根节点 /// private void BindRootNode() { TreeNode tn = new TreeNode(); tn.Tag = 0; //绑定隐藏数据 tn.Text = "全部商品"; tvDemo.Nodes.Add(tn); //将创建出来的节点添加到TreeView控件中 BindGoodsType(tn); //绑定商品分类,将商品分类添加到"全部商品"节点中 } /// /// 加载商品分类 /// /// 根节点 private void BindGoodsType(TreeNode tn) { // 根节点创建后,绑定一级目录 [商品分类] string getGoodsTypeInfo = $"SELECT TID,TypeName FROM GoodsType"; SqlDataReader r = DBHelper.ExecuteReader(getGoodsTypeInfo); while (r != null && r.HasRows && r.Read()) { TreeNode typeNode = new TreeNode(); typeNode.Text = r["TypeName"].ToString(); typeNode.Tag = r["TID"]; tn.Nodes.Add(typeNode); // 在绑定该分类后,立马绑定当前分类下所有商品信息 BindGoodsInfo(typeNode); } if (r != null) r.Close(); } /// /// 加载指定分类的商品信息 /// /// 商品分类节点 private void BindGoodsInfo(TreeNode typeNode) { string getGoodsTypeInfo = $"SELECT GID,GoodsName,Price FROM Goods WHERE TID = {typeNode.Tag}"; SqlDataReader r = DBHelper.ExecuteReader(getGoodsTypeInfo); while (r != null && r.HasRows && r.Read()) { TreeNode goodsNode = new TreeNode(); goodsNode.Text = $"{r["GoodsName"]} - {r["Price"]}元"; goodsNode.Tag = r["GID"]; typeNode.Nodes.Add(goodsNode); } if (r != null) r.Close(); } } } ``` [scode type="blue" size="simple"] 如果需要给指定的节点绑定图片,可以将图片存储至: `ImageList`控件 可以使用如下代码将 `ImageList`控件绑定给 `ListView`控件: `tvDemo.ImageList = imglDemo; //imglDemo是ImageList控件的名称` 可以使用如下代码指定 `TreeView`节点显示图片(`tn`是`TreeNode`节点): `tn.ImageIndex = 1; //绑定节点默认状态时显示的图片` `tn.SelectedImageIndex = 0; //绑定节点选中时需要显示的图片` [/scode] Loading... ## 数据表结构及关系  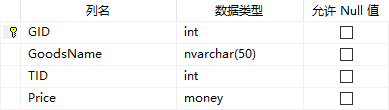 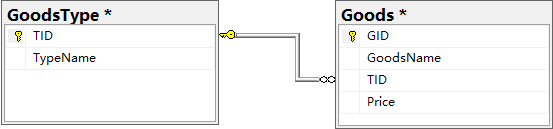 ## 创建数据库帮助类 ```csharp using System; using System.Data; using System.Data.SqlClient; using System.Windows.Forms; namespace TreeViewDemo { /// <summary> /// 数据库帮助类 /// </summary> public static class DBHelper { /// <summary> /// 连接字符串 /// </summary> private static string constr = @"server=.;database=Demo;user=sa;pwd=cssl#123"; /// <summary> /// 获取读取器,使用完毕时需要关闭读取器 /// </summary> /// <param name="sql">需要执行的SQL语句</param> /// <returns>数据读取器</returns> public static SqlDataReader ExecuteReader(string sql) { // 创建数据库连接对象,并指定连接字符串 SqlConnection con = new SqlConnection(constr); // 创建命令对象 SqlCommand cmd = new SqlCommand(sql, con); try { con.Open(); return cmd.ExecuteReader(CommandBehavior.CloseConnection); } catch (Exception ex) { MessageBox.Show($"访问数据库是发生意外,错误信息:\r\r{ex.Message}", "温馨提示", MessageBoxButtons.OK, MessageBoxIcon.Error); } return null; } } } ``` ## 窗体加载时,创建默认根节点 ```csharp /// <summary> /// 窗体加载时触发该事件 /// </summary> /// <param name="sender"></param> /// <param name="e"></param> private void frmTreeViewDmeo_Load(object sender, EventArgs e) { BindRootNode(); //绑定根节点 tvDemo.ExpandAll(); //展开所有节点 } /// <summary> /// 绑定根节点 /// </summary> private void BindRootNode() { TreeNode tn = new TreeNode(); tn.Tag = 0; //绑定隐藏数据 tn.Text = "全部商品"; tvDemo.Nodes.Add(tn); //将创建出来的节点添加到TreeView控件中 } ``` 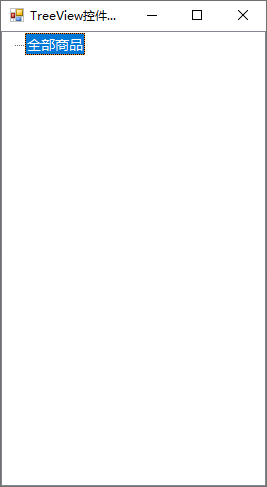 ## 绑定二级节点-商品分类 在 `BindRootNode()`方法最末尾添加如下代码: ```csharp BindGoodsType(tn); //绑定商品分类,将商品分类添加到"全部商品"节点中 ``` `BindGoodsType(TreeNode tn)`方法定义如下: ```csharp /// <summary> /// 加载商品分类 /// </summary> /// <param name="tn">根节点</param> private void BindGoodsType(TreeNode tn) { // 根节点创建后,绑定一级目录 [商品分类] string getGoodsTypeInfo = $"SELECT TID,TypeName FROM GoodsType"; SqlDataReader r = DBHelper.ExecuteReader(getGoodsTypeInfo); while (r != null && r.HasRows && r.Read()) { TreeNode typeNode = new TreeNode(); typeNode.Text = r["TypeName"].ToString(); typeNode.Tag = r["TID"]; tn.Nodes.Add(typeNode); } if (r != null) r.Close(); } ``` 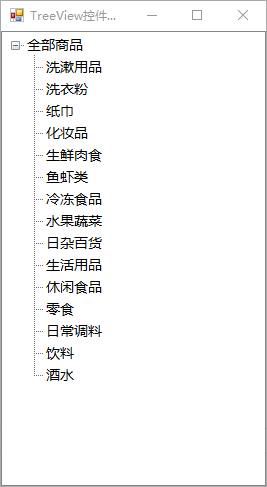 ## 加载不同分类下的不同商品信息 在方法 `BindGoodsType(TreeNode tn)`里的 `while`循环中最末尾添加如下代码: ```csharp // 在绑定该分类后,立马绑定当前分类下所有商品信息 BindGoodsInfo(typeNode); ``` `BindGoodsInfo(TreeNode typeNode)`方法定义如下: ```csharp /// <summary> /// 加载指定分类的商品信息 /// </summary> /// <param name="typeNode">商品分类节点</param> private void BindGoodsInfo(TreeNode typeNode) { string getGoodsTypeInfo = $"SELECT GID,GoodsName,Price FROM Goods WHERE TID = {typeNode.Tag}"; SqlDataReader r = DBHelper.ExecuteReader(getGoodsTypeInfo); while (r != null && r.HasRows && r.Read()) { TreeNode goodsNode = new TreeNode(); goodsNode.Text = $"{r["GoodsName"]} - {r["Price"]}元"; goodsNode.Tag = r["GID"]; typeNode.Nodes.Add(goodsNode); } if (r != null) r.Close(); } ``` 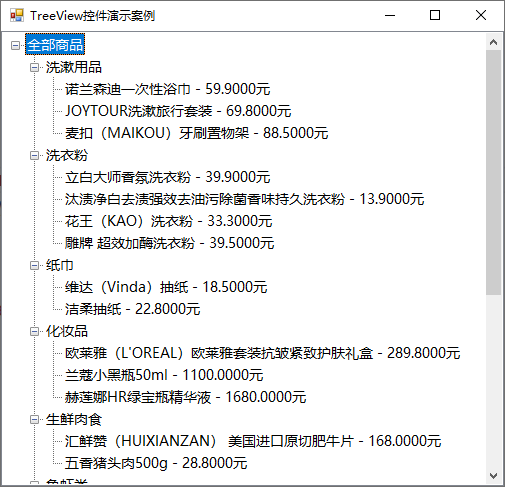 ## 完整代码展示 ```csharp using System; using System.Windows.Forms; using System.Data.SqlClient; namespace TreeViewDemo { public partial class frmTreeViewDmeo : Form { public frmTreeViewDmeo() { InitializeComponent(); } /// <summary> /// 窗体加载时触发该事件 /// </summary> /// <param name="sender"></param> /// <param name="e"></param> private void frmTreeViewDmeo_Load(object sender, EventArgs e) { BindRootNode(); //绑定根节点 tvDemo.ExpandAll(); //展开所有节点 } /// <summary> /// 绑定根节点 /// </summary> private void BindRootNode() { TreeNode tn = new TreeNode(); tn.Tag = 0; //绑定隐藏数据 tn.Text = "全部商品"; tvDemo.Nodes.Add(tn); //将创建出来的节点添加到TreeView控件中 BindGoodsType(tn); //绑定商品分类,将商品分类添加到"全部商品"节点中 } /// <summary> /// 加载商品分类 /// </summary> /// <param name="tn">根节点</param> private void BindGoodsType(TreeNode tn) { // 根节点创建后,绑定一级目录 [商品分类] string getGoodsTypeInfo = $"SELECT TID,TypeName FROM GoodsType"; SqlDataReader r = DBHelper.ExecuteReader(getGoodsTypeInfo); while (r != null && r.HasRows && r.Read()) { TreeNode typeNode = new TreeNode(); typeNode.Text = r["TypeName"].ToString(); typeNode.Tag = r["TID"]; tn.Nodes.Add(typeNode); // 在绑定该分类后,立马绑定当前分类下所有商品信息 BindGoodsInfo(typeNode); } if (r != null) r.Close(); } /// <summary> /// 加载指定分类的商品信息 /// </summary> /// <param name="typeNode">商品分类节点</param> private void BindGoodsInfo(TreeNode typeNode) { string getGoodsTypeInfo = $"SELECT GID,GoodsName,Price FROM Goods WHERE TID = {typeNode.Tag}"; SqlDataReader r = DBHelper.ExecuteReader(getGoodsTypeInfo); while (r != null && r.HasRows && r.Read()) { TreeNode goodsNode = new TreeNode(); goodsNode.Text = $"{r["GoodsName"]} - {r["Price"]}元"; goodsNode.Tag = r["GID"]; typeNode.Nodes.Add(goodsNode); } if (r != null) r.Close(); } } } ``` <div class="tip inlineBlock info simple"> 如果需要给指定的节点绑定图片,可以将图片存储至: `ImageList`控件 可以使用如下代码将 `ImageList`控件绑定给 `ListView`控件: `tvDemo.ImageList = imglDemo; //imglDemo是ImageList控件的名称` 可以使用如下代码指定 `TreeView`节点显示图片(`tn`是`TreeNode`节点): `tn.ImageIndex = 1; //绑定节点默认状态时显示的图片` `tn.SelectedImageIndex = 0; //绑定节点选中时需要显示的图片` </div> 最后修改:2023 年 04 月 27 日 © 允许规范转载 赞 都滑到这里了,不点赞再走!?